In my last few Kamailio Bytes posts, I’ve talked about using the GeoIP2 module to lookup the location of IP Addresses and SQLops and db_mysql to work with relational databases from within Kamailio.
Now we’ll put both together to create something functional you could use in your own deployments. (You’d often find it’s faster to use HTable to store and retrieve data like this, but that’s a conversation for another day)
The Project
We’ll build a SIP honeypot using Kamailio. It’ll listen on a Public IP address for SIP connections from people scanning the internet with malicious intent and log their IPs, so our real SIP softswitches know to ignore them.
We’ll use GeoIP2 to lookup the location of the IP and then store that data into a MySQL database.
Lastly we’ll create a routing block we can use on another Kamailio instance to verify if that the IP address of the received SIP message is not in our blacklist by searching the MySQL database for the source IP.
The Database
In this example I’m going to create a database called “blacklist” with one table called “baddies”, in MySQL I’ll run:
CREATE database blacklist;
CREATE TABLE `baddies` (
`id` INT NOT NULL AUTO_INCREMENT PRIMARY KEY,
`ip_address` INT unsigned UNIQUE,
`hits` INT,
`last_seen` DATETIME,
`ua` TEXT,
`country` TEXT,
`city` TEXT
);
I’ll setup a MySQL user to INSERT/UPDATE/SELECT data from the MySQL database.
For storing IP addresses in the database we’ll store them as unsigned integers, and then use the INET_ATON('127.0.0.1')
MySQL command to encode them from dotted-decimal format, and the INET_NTOA('2130706433')
to put them back into dotted decimal.
Modparams
Now we’ll need to configure Kamailio, I’ll continue on from where we left off in the last post on GeoIP2 as we’ll use that to put Geographic data about the IP before adding the MySQL and SQLOps modules:
# ----- SQL params -----
loadmodule "db_mysql.so"
loadmodule "sqlops.so"
#Create a new MySQL database connection called blacklist_db
modparam("sqlops","sqlcon","blacklist_db=>mysql://root:yourpassword@localhost/blacklist")
#Set timeouts for MySQL Connections
modparam("db_mysql", "ping_interval", 60)
modparam("db_mysql", "auto_reconnect", 1)
modparam("db_mysql", "timeout_interval", 2)
After loading db_mysql and sqlops we create a new object / connection called blacklist_db with our MySQL Database parameters.
Now after a restart we’ll be connected to our MySQL database.
Honeypot Routing Logic
Now we’ll create a route to log the traffic:
####### Routing Logic ########
/* Main SIP request routing logic
* - processing of any incoming SIP request starts with this route
* - note: this is the same as route { ... } */
request_route {
route(AddToBlacklist);
sl_reply('200', 'Sure thing boss!');
}
route[AddToBlacklist]{
xlog("Packet received from IP $si");
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (2130706433, 10, NOW(), 'testua2', 'Australia', 'Hobart');");
}
Now for each SIP message received a new record will be inserted into the database:
root@ip-172-31-8-156:/etc/kamailio# mysql -u root -p blacklist -e "select * from baddies;" Enter password: +----+------------+------+---------------------+---------+-----------+--------+ | id | ip_address | hits | last_seen | ua | country | city | +----+------------+------+---------------------+---------+-----------+--------+ | 1 | 2130706433 | 10 | 2019-08-13 02:52:57 | testua2 | Australia | Hobart | | 2 | 2130706433 | 10 | 2019-08-13 02:53:01 | testua2 | Australia | Hobart | | 3 | 2130706433 | 10 | 2019-08-13 02:53:05 | testua2 | Australia | Hobart | +----+------------+------+---------------------+---------+-----------+--------+
This is great but we’re not actually putting the call variables in here, and we’ve got a lot of duplicates, let’s modify our sql_xquery() to include the call variables:
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (INET_ATON('$si'), 10, NOW(), '$ua', 'Australia', 'Hobart');");
Now we’re setting the IP Address value to the Source IP psedovariable ($si) and formatting it using the INET_ATON function in MySQL, setting the last_seen to the current timestamp and setting the user agent to the User Agent psedovariable ($ua).
Let’s restart Kamailio, truncate the data that’s currently in the DB, send some SIP traffic to it and then check the contents:
mysql -u root -p blacklist -e "select *, INET_NTOA(ip_address) from baddies;"

Here you can see we’re starting to get somewhere, the IP, UA and last_seen values are all now correct.
We’re getting multiple entries from the same IP though, instead we just want to increment the hits counter and set the last_seen to the current time, for that we’ll just update the SQL query to set the time to be NOW() and if that IP is already in the database to update the last_seen value and incriment the hits counter:
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (INET_ATON('$si'), 1, NOW(), '$ua', 'Australia', 'Hobart') ON DUPLICATE KEY UPDATE last_seen = NOW(), hits = hits + 1;");
Now we’ve only got one line per IP address, with the hit counters showing how many SIP requests we’ve received from that IP.
Finally we’ll use the GeoIP2 Module to get the geographic info of the source of the traffic, like we’ve talked about in the previous post:
route[AddToBlacklist]{
xlog("Packet received from IP $si");
geoip2_match("$si", "src"))
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (INET_ATON('$si'), 1, NOW(), '$ua', '$gip2(src=>cc)', '$gip2(src=>city)') ON DUPLICATE KEY UPDATE last_seen = NOW(), hits = hits + 1;", "r_sql");
}
The only issue with this is if GeoIP2 doesn’t have a match, no record will be added in the database, so we’ll add a handler for that:
route[AddToBlacklist]{
xlog("Packet received from IP $si");
if(geoip2_match("$si", "src")){
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (INET_ATON('$si'), 1, NOW(), '$ua', '$gip2(src=>cc)', '$gip2(src=>city)') ON DUPLICATE KEY UPDATE last_seen = NOW(), hits = hits + 1;", "r_sql");
}else{ ##If no match in GeoIP2 leave Country & City fields blank
sql_xquery("blacklist_db", "insert into baddies (ip_address, hits, last_seen, ua, country, city) values (INET_ATON('$si'), 1, NOW(), '$ua', '', '') ON DUPLICATE KEY UPDATE last_seen = NOW(), hits = hits + 1;", "r_sql");
}
}
Now let’s check our database again and see how the data looks:
mysql -u root -p blacklist -e "select *, INET_NTOA(ip_address) from baddies;"

Perfect! Now we’re inserting data into our blacklist from our honeypot. Now we’ll configure a new routing block we can use on another Kamailio instance to see if an IP is in the blacklist.
I left this running on my AWS box for a few hours, and lots of dodgy UAs dropped in to say hello, one of which was very insistent on making calls to Poland…
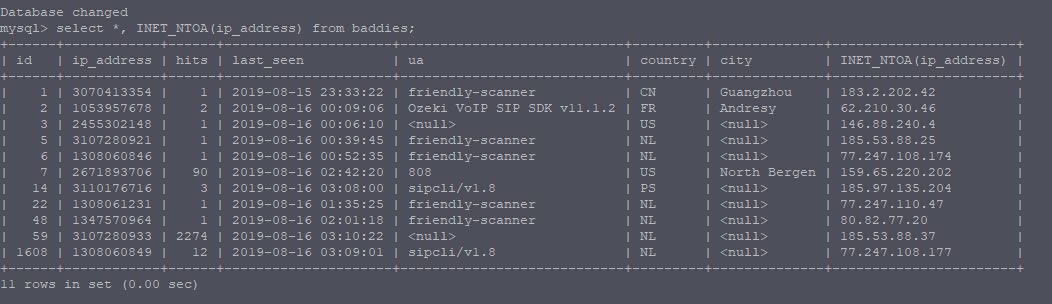
Querying the Data
Now we’ve got a blacklist it’s only useful if we block the traffic from our malicous actors who we’ve profiled in the database.
You could feed this into BGP to null route the traffic, or hook this into your firewall’s API, but we’re going to do this in Kamailio, so we’ll create a new routing block we can use on a different Kamailio instance – Like a production one – to see if the IP it just received traffic from is in the blacklist.
We’ve already spoken about querying databases in the SQLops Kamailio bytes, but this routing block will query the blacklist database, and if the sender is in the database, one or more records will be returned, so we know they’re bad and will drop their traffic:
route[CheckBlacklist]{
xlog("Checking blacklist for ip $si");
#Define a variable containing the SQL query we'll run
$var(sql) = "select INET_NTOA(ip_address) as ip_address from baddies;";
#Log the SQL query we're going to run to syslog for easy debugging
xlog("Query to run is $var(sql)");
#Query blacklist_db running the query stored in $var(sql) and store the result of the query to result_sql
sql_query("blacklist_db", "$var(sql)", "result_sql");
#If more than 0 records were returned from the database, drop the traffic
if($dbr(result_sql=>rows)>0){
xlog("This guy is bad news. Dropping traffic from $si");
exit;
}else{
xlog("No criminal record for $si - Allowing to progress");
}
}
Recap
We’d previously touched on using the GeoIP2 module to lookup the location of IP Addresses and SQLops and db_mysql to work with relational databases from within Kamailio.
This Honeypot use case just put those elements together.
In reality a far better implementation of this would use HTable to store this data, but hopefully this gives you a better understanding of how to actually work with data.
Final Note
I wrote this post about a week ago, and left the config running on an AWS box. I was getting hits to it within the hour, and in the past week I’ve had 172 IPs come and say hello, and some like the FriendlyScanner instance at 159.65.220.215 has sent over 93,000 requests:
mysql> select *, INET_NTOA(ip_address) from baddies; +--------+------------+-------+---------------------+----------------------------+---------+---------------------+-----------------------+ | id | ip_address | hits | last_seen | ua | country | city | INET_NTOA(ip_address) | +--------+------------+-------+---------------------+----------------------------+---------+---------------------+-----------------------+ | 1 | 3070413354 | 23 | 2019-08-23 14:15:40 | friendly-scanner | CN | Guangzhou | 183.2.202.42 | | 2 | 1053957678 | 2 | 2019-08-16 00:09:06 | Ozeki VoIP SIP SDK v11.1.2 | FR | Andresy | 62.210.30.46 | | 3 | 2455302148 | 7 | 2019-08-23 00:05:03 | | US | | 146.88.240.4 | | 5 | 3107280921 | 26 | 2019-08-23 20:46:59 | friendly-scanner | NL | | 185.53.88.25 | | 6 | 1308060846 | 53 | 2019-08-23 20:13:12 | friendly-scanner | NL | | 77.247.108.174 | | 7 | 2671893706 | 90 | 2019-08-16 02:42:20 | 808 | US | North Bergen | 159.65.220.202 | | 14 | 3110176716 | 6 | 2019-08-16 04:44:25 | sipcli/v1.8 | PS | | 185.97.135.204 | | 22 | 1308061231 | 18 | 2019-08-22 07:24:38 | friendly-scanner | NL | | 77.247.110.47 | | 48 | 1347570964 | 8 | 2019-08-17 02:24:36 | friendly-scanner | NL | | 80.82.77.20 | | 59 | 3107280933 | 9883 | 2019-08-19 03:03:32 | | NL | | 185.53.88.37 | | 1608 | 1308060849 | 35 | 2019-08-16 03:59:29 | sipcli/v1.8 | NL | | 77.247.108.177 | | 3967 | 3428686544 | 1 | 2019-08-16 04:03:13 | Asterisk PBX | US | Chicago | 204.93.154.208 | | 3994 | 1754805254 | 1 | 2019-08-16 04:03:52 | Asterisk PBX | US | | 104.152.52.6 | | 5290 | 3507576938 | 1 | 2019-08-16 04:37:12 | UnYeznuK | US | | 209.17.96.106 | | 7905 | 3107280927 | 3867 | 2019-08-22 11:42:00 | | NL | | 185.53.88.31 | | 8012 | 1356068398 | 1 | 2019-08-16 05:52:59 | Zeeko | PL | Warsaw | 80.211.246.46 | | 8207 | 3107280926 | 1825 | 2019-08-21 06:01:23 | | NL | | 185.53.88.30 | | 8412 | 3070413353 | 10 | 2019-08-23 07:18:04 | friendly-scanner | CN | Guangzhou | 183.2.202.41 | | 8415 | 1308060851 | 1 | 2019-08-16 06:13:32 | friendly-scanner | NL | | 77.247.108.179 | | 8746 | 1308060835 | 31 | 2019-08-16 08:45:51 | sipcli/v1.8 | NL | | 77.247.108.163 | | 8755 | 3107280940 | 3 | 2019-08-17 16:21:55 | friendly-scanner | NL | | 185.53.88.44 | | 8761 | 1111941599 | 1 | 2019-08-16 07:26:55 | friendly-scanner | CA | Montreal | 66.70.225.223 | | 9009 | 1308060832 | 9 | 2019-08-18 00:22:01 | friendly-scanner | NL | | 77.247.108.160 | | 9250 | 2746007762 | 805 | 2019-08-20 10:26:04 | pplsip | FR | | 163.172.192.210 | | 9440 | 1308061242 | 16 | 2019-08-23 21:10:59 | friendly-scanner | NL | | 77.247.110.58 | | 9458 | 3635051022 | 328 | 2019-08-17 11:17:00 | pplsip | US | Buffalo | 216.170.122.14 | | 9474 | 2959836555 | 8 | 2019-08-23 09:27:08 | Bria 5 | PL | Warsaw | 176.107.133.139 | | 9494 | 3107280945 | 7 | 2019-08-22 18:24:01 | friendly-scanner | NL | | 185.53.88.49 | | 9516 | 1308061267 | 64 | 2019-08-23 19:38:43 | friendly-scanner | NL | | 77.247.110.83 | | 9546 | 1308061199 | 1884 | 2019-08-17 00:19:02 | sipcli/v1.8 | NL | | 77.247.110.15 | | 9684 | 1308061400 | 7 | 2019-08-23 20:59:58 | friendly-scanner | NL | | 77.247.110.216 | | 10088 | 3565227944 | 2 | 2019-08-22 17:47:04 | friendly-scanner | FR | | 212.129.15.168 | | 10335 | 1588721057 | 3 | 2019-08-19 12:08:11 | friendly-scanner | DE | Frankfurt am Main | 94.177.245.161 | | 10372 | 3107280931 | 8 | 2019-08-21 00:32:14 | friendly-scanner | NL | | 185.53.88.35 | | 10440 | 1588717692 | 6 | 2019-08-18 11:37:20 | friendly-scanner | FR | Paris | 94.177.232.124 | | 10451 | 3507577090 | 1 | 2019-08-16 18:18:32 | haLoBtKl | US | | 209.17.97.2 | | 10507 | 1505473210 | 1 | 2019-08-16 18:33:49 | pplsip | US | | 89.187.178.186 | | 10653 | 3562251402 | 90 | 2019-08-22 17:24:00 | pplsip | FR | Nuits-Saint-Georges | 212.83.164.138 | | 11077 | 861095860 | 1 | 2019-08-16 21:11:03 | friendly-scanner | FR | | 51.83.71.180 | | 11157 | 1722098888 | 197 | 2019-08-22 14:11:49 | sipcli/v1.8 | US | | 102.165.36.200 | | 11408 | 87965623 | 37 | 2019-08-17 12:18:29 | pplsip | US | New York | 5.62.63.183 | | 11589 | 1308061418 | 5 | 2019-08-19 12:34:55 | Telefonadapter | NL | | 77.247.110.234 | | 11781 | 3281662327 | 3 | 2019-08-20 14:51:30 | support | FR | Paris | 195.154.49.119 | | 11812 | 2680904312 | 1 | 2019-08-17 01:09:40 | friendly-scanner | US | Clifton | 159.203.90.120 | | 11826 | 624027040 | 258 | 2019-08-23 21:31:43 | Telefonadapter | NL | | 37.49.229.160 | | 11827 | 1051561183 | 1 | 2019-08-17 01:47:04 | friendly-scanner | RU | | 62.173.140.223 | | 11830 | 3514713984 | 3 | 2019-08-18 02:57:23 | sipcli/v1.8 | US | St Louis | 209.126.71.128 | | 11863 | 2382633440 | 1 | 2019-08-17 03:22:02 | friendly-scanner | US | Provo | 142.4.25.224 | | 11895 | 1123085431 | 1 | 2019-08-17 05:05:58 | | US | San Diego | 66.240.236.119 | | 11917 | 3562247345 | 1 | 2019-08-17 05:58:21 | Bria 5 | FR | Paris | 212.83.148.177 | | 11951 | 3507577002 | 1 | 2019-08-17 07:18:45 | vCbNSKtv | US | | 209.17.96.170 | | 11997 | 2256000670 | 2 | 2019-08-18 22:15:28 | friendly-scanner | FR | | 134.119.214.158 | | 12012 | 2806494445 | 1 | 2019-08-17 09:08:55 | friendly-scanner | US | New York | 167.71.180.237 | | 12060 | 1588719724 | 12958 | 2019-08-17 10:34:45 | Asterisk PBX | FR | Paris | 94.177.240.108 | | 25027 | 1308060847 | 3 | 2019-08-17 14:05:12 | PortSIP VoIP SDK 11.2 | NL | | 77.247.108.175 | | 25052 | 1051560788 | 6 | 2019-08-22 23:41:20 | friendly-scanner | RU | | 62.173.139.84 | | 25061 | 2769743287 | 3827 | 2019-08-20 13:28:17 | | CA | Toronto | 165.22.237.183 | | 25089 | 624027025 | 237 | 2019-08-23 21:30:21 | Cisco-SIPGateway/IOS-12.x | NL | | 37.49.229.145 | | 25135 | 1308060845 | 1 | 2019-08-17 13:13:26 | friendly-scanner | NL | | 77.247.108.173 | | 25314 | 1356068336 | 4 | 2019-08-23 06:41:51 | | PL | Warsaw | 80.211.245.240 | | 25342 | 1308061206 | 5 | 2019-08-21 05:50:11 | friendly-scanner | NL | | 77.247.110.22 | | 27328 | 3507576890 | 2 | 2019-08-19 20:41:58 | BQDPtxsE | US | | 209.17.96.58 | | 28414 | 3107280932 | 1 | 2019-08-17 22:24:17 | friendly-scanner | NL | | 185.53.88.36 | | 29304 | 2745967553 | 2 | 2019-08-21 12:05:24 | friendly-scanner | FR | | 163.172.35.193 | | 29317 | 2671893719 | 93202 | 2019-08-18 01:55:38 | friendly-scanner | US | North Bergen | 159.65.220.215 | | 122638 | 624634839 | 1 | 2019-08-18 03:44:42 | Ozeki VoIP SIP SDK v11.1.2 | FR | | 37.59.43.215 | | 122643 | 1308060935 | 1 | 2019-08-18 03:48:16 | | NL | | 77.247.109.7 | | 122692 | 3107280934 | 8470 | 2019-08-18 15:22:11 | | NL | | 185.53.88.38 | | 123190 | 1308061276 | 2 | 2019-08-22 08:42:06 | friendly-scanner | NL | | 77.247.110.92 | | 123505 | 860848595 | 1 | 2019-08-18 05:28:07 | friendly-scanner | CA | | 51.79.129.211 | | 124707 | 3565236782 | 3 | 2019-08-19 22:04:23 | Careers | FR | Paris | 212.129.50.46 | | 130299 | 3119862842 | 1 | 2019-08-18 12:51:44 | VOIP | DK | Copenhagen | 185.245.84.58 | | 130398 | 394281064 | 1 | 2019-08-18 13:00:27 | friendly-scanner | US | Portland | 23.128.64.104 | | 131093 | 3644653804 | 2 | 2019-08-20 08:21:00 | friendly-scanner | DE | Frankfurt am Main | 217.61.0.236 | | 131760 | 1588720143 | 1 | 2019-08-18 15:09:48 | friendly-scanner | FR | Paris | 94.177.242.15 | | 131891 | 3633377286 | 1 | 2019-08-18 16:03:16 | friendly-scanner | US | Dallas | 216.144.240.6 | | 131905 | 3510351397 | 12 | 2019-08-23 14:24:14 | PortSIP VoIP SDK 11.2 | US | Lansing | 209.59.182.37 | | 131979 | 1356070905 | 1 | 2019-08-18 17:10:47 | friendly-scanner | PL | Warsaw | 80.211.255.249 | | 132142 | 1308060872 | 1 | 2019-08-18 19:27:15 | friendly-scanner | NL | | 77.247.108.200 | | 132163 | 3507577122 | 1 | 2019-08-18 19:45:08 | jXIjYZTm | US | | 209.17.97.34 | | 132185 | 1308061207 | 2 | 2019-08-19 20:21:17 | friendly-scanner | NL | | 77.247.110.23 | | 132244 | 1431941221 | 2 | 2019-08-22 03:46:37 | friendly-scanner | PL | �ódź | 85.89.176.101 | | 133058 | 1308061211 | 1 | 2019-08-19 00:03:59 | PBX | NL | | 77.247.110.27 | | 133193 | 861606239 | 1 | 2019-08-19 00:27:24 | friendly-scanner | FR | | 51.91.17.95 | | 133194 | 2261891524 | 1 | 2019-08-19 00:27:26 | friendly-scanner | GB | London | 134.209.185.196 | | 133247 | 1311362863 | 1 | 2019-08-19 00:35:16 | friendly-scanner | NL | | 78.41.207.47 | | 133562 | 3221485468 | 4 | 2019-08-23 00:50:28 | friendly-scanner | US | Buffalo | 192.3.247.156 | | 133573 | 2673412367 | 1 | 2019-08-19 01:28:30 | friendly-scanner | DE | Frankfurt am Main | 159.89.9.15 | | 134160 | 861605605 | 690 | 2019-08-19 12:20:33 | gffg | FR | | 51.91.14.229 | | 134218 | 3562255759 | 1 | 2019-08-19 05:15:30 | friendly-scanner | FR | | 212.83.181.143 | | 134412 | 1053973250 | 22 | 2019-08-23 19:24:58 | friendly-scanner | FR | Paris | 62.210.91.2 | | 134556 | 1308061221 | 1 | 2019-08-19 08:19:53 | friendly-scanner | NL | | 77.247.110.37 | | 134665 | 1249543663 | 1 | 2019-08-19 10:06:49 | friendly-scanner | CA | | 74.122.133.239 | | 134750 | 93785829 | 1 | 2019-08-19 10:52:57 | friendly-scanner | GB | | 5.151.14.229 | | 134831 | 1347570977 | 1 | 2019-08-19 11:36:12 | | NL | | 80.82.77.33 | | 134939 | 3225441884 | 3 | 2019-08-23 12:53:33 | friendly-scanner | US | Secaucus | 192.64.86.92 | | 134944 | 3276722437 | 1 | 2019-08-19 14:48:33 | friendly-scanner | IT | | 195.78.209.5 | | 134945 | 1122091794 | 147 | 2019-08-19 15:48:28 | MizuPhone | US | Schaumburg | 66.225.195.18 | | 135140 | 3000185825 | 1 | 2019-08-19 19:01:47 | friendly-scanner | TR | Samsun | 178.211.51.225 | | 135169 | 2745980561 | 1 | 2019-08-19 20:38:42 | friendly-scanner | FR | | 163.172.86.145 | | 135205 | 1066345778 | 77 | 2019-08-23 06:04:18 | FreePBX 1.8 | US | Dallas | 63.143.37.50 | | 135212 | 1053994118 | 1 | 2019-08-19 22:28:37 | friendly-scanner | FR | | 62.210.172.134 | | 135218 | 3565239451 | 2 | 2019-08-19 22:49:18 | VaxSIPUserAgent/3.5 | FR | Le Plessis-Robinson | 212.129.60.155 | | 135225 | 3639984622 | 3 | 2019-08-22 20:40:42 | friendly-scanner | US | Dallas | 216.245.193.238 | | 135246 | 3000185822 | 1 | 2019-08-19 23:56:45 | friendly-scanner | TR | Samsun | 178.211.51.222 | | 135266 | 3562255783 | 1 | 2019-08-20 00:42:30 | friendly-scanner | FR | | 212.83.181.167 | | 135284 | 1308061283 | 2 | 2019-08-22 06:51:28 | friendly-scanner | NL | | 77.247.110.99 | | 135289 | 2727356702 | 1 | 2019-08-20 01:31:33 | friendly-scanner | US | Provo | 162.144.41.30 | | 135324 | 1053992906 | 6758 | 2019-08-23 00:25:28 | fgfdhgfxjfhyjhkj | FR | | 62.210.167.202 | | 135353 | 1053988629 | 4944 | 2019-08-23 00:25:51 | fgfdhgfxjfhyjhkj | FR | | 62.210.151.21 | | 135695 | 782669768 | 5 | 2019-08-20 04:15:47 | fgfdhgfxjfhyjhkj | NL | Uddel | 46.166.151.200 | | 136185 | 782669779 | 35927 | 2019-08-22 20:33:51 | fgfdhgfxjfhyjhkj | NL | Uddel | 46.166.151.211 | | 138056 | 3562244647 | 2 | 2019-08-21 06:56:33 | testsip | FR | Guyancourt | 212.83.138.39 | | 141492 | 3281660074 | 2 | 2019-08-23 03:57:42 | Bria 5 | FR | Argenteuil | 195.154.40.170 | | 141905 | 2649154441 | 2025 | 2019-08-20 15:24:27 | | US | North Bergen | 157.230.227.137 | | 142082 | 3107280950 | 1 | 2019-08-20 11:55:44 | friendly-scanner | NL | | 185.53.88.54 | | 144875 | 3107280956 | 2 | 2019-08-23 03:08:10 | friendly-scanner | NL | | 185.53.88.60 | | 144881 | 3281677282 | 74 | 2019-08-20 23:27:29 | | FR | | 195.154.107.226 | | 148332 | 3423277731 | 1 | 2019-08-20 17:15:49 | friendly-scanner | US | Seattle | 204.11.18.163 | | 148463 | 866041843 | 1 | 2019-08-20 17:29:43 | friendly-scanner | FR | Paris | 51.158.191.243 | | 148935 | 3000019324 | 1 | 2019-08-20 18:17:37 | friendly-scanner | GB | London | 178.208.169.124 | | 150006 | 1051562889 | 1 | 2019-08-20 20:02:47 | friendly-scanner | RU | Moscow | 62.173.147.137 | | 150459 | 1308060874 | 4 | 2019-08-21 23:39:45 | friendly-scanner | NL | | 77.247.108.202 | | 151158 | 3291753334 | 1 | 2019-08-20 22:05:46 | VhPrwfzK | US | Edison | 196.52.43.118 | | 152525 | 1308061269 | 1 | 2019-08-21 00:31:18 | friendly-scanner | NL | | 77.247.110.85 | | 152748 | 1588719753 | 2 | 2019-08-21 20:19:51 | friendly-scanner | FR | Paris | 94.177.240.137 | | 152938 | 1308061244 | 862 | 2019-08-22 16:25:35 | | NL | | 77.247.110.60 | | 154063 | 3639886154 | 216 | 2019-08-22 02:36:47 | sipcli/v1.8 | US | Seattle | 216.244.65.74 | | 154100 | 2806460368 | 1 | 2019-08-21 02:06:05 | friendly-scanner | DE | Frankfurt am Main | 167.71.47.208 | | 155564 | 1308061232 | 430 | 2019-08-21 04:50:00 | | NL | | 77.247.110.48 | | 156748 | 3107280962 | 1 | 2019-08-21 04:32:50 | friendly-scanner | NL | | 185.53.88.66 | | 158028 | 3507576914 | 1 | 2019-08-21 05:49:21 | yMQsDpTp | US | | 209.17.96.82 | | 159649 | 2659999515 | 3 | 2019-08-21 11:24:18 | PortSIP VoIP SDK 11.2 | PS | | 158.140.95.27 | | 159710 | 2769728503 | 1 | 2019-08-21 09:12:10 | 808 | US | North Bergen | 165.22.179.247 | | 160120 | 2755949120 | 1 | 2019-08-21 09:53:12 | friendly-scanner | DE | | 164.68.114.64 | | 160495 | 2809328307 | 1 | 2019-08-21 10:31:39 | friendly-scanner | FR | Roubaix | 167.114.242.179 | | 160763 | 2807459643 | 2 | 2019-08-23 19:48:17 | friendly-scanner | DE | Nuremberg | 167.86.111.59 | | 161033 | 1356048200 | 1 | 2019-08-21 11:27:02 | friendly-scanner | IT | Arezzo | 80.211.167.72 | | 162648 | 1245704902 | 1 | 2019-08-21 14:08:05 | friendly-scanner | US | Dallas | 74.63.242.198 | | 163133 | 1308060834 | 3 | 2019-08-23 06:01:53 | friendly-scanner | NL | | 77.247.108.162 | | 168263 | 2746011496 | 552 | 2019-08-23 14:00:56 | pplsip | FR | | 163.172.207.104 | | 168773 | 2807450735 | 1 | 2019-08-21 21:25:23 | friendly-scanner | DE | Nuremberg | 167.86.76.111 | | 169044 | 1308061297 | 17 | 2019-08-23 21:17:33 | FreePBX 1.8 | NL | | 77.247.110.113 | | 169067 | 3507577202 | 1 | 2019-08-21 21:44:59 | YtsNMLcO | US | | 209.17.97.114 | | 171542 | 1588717792 | 1 | 2019-08-22 00:23:29 | friendly-scanner | FR | Paris | 94.177.232.224 | | 171799 | 1053987565 | 12958 | 2019-08-22 00:42:46 | Asterisk PBX | FR | | 62.210.146.237 | | 185012 | 1334405281 | 2 | 2019-08-22 00:56:21 | VaxSIPUserAgent/3.5 | FR | | 79.137.104.161 | | 185327 | 87965663 | 25 | 2019-08-22 07:07:17 | pplsip | US | New York | 5.62.63.223 | | 187784 | 1090198397 | 2 | 2019-08-23 05:41:24 | friendly-scanner | US | Fort Lauderdale | 64.251.27.125 | | 187866 | 2731950313 | 12958 | 2019-08-22 04:06:58 | Asterisk PBX | US | Provo | 162.214.64.233 | | 202877 | 3107280937 | 1 | 2019-08-22 06:18:42 | friendly-scanner | NL | | 185.53.88.41 | | 206150 | 3221475752 | 2 | 2019-08-23 05:25:06 | friendly-scanner | US | Buffalo | 192.3.209.168 | | 207013 | 3106407447 | 2 | 2019-08-22 10:45:26 | MizuPhone | RU | | 185.40.4.23 | | 207253 | 3537074702 | 1 | 2019-08-22 11:00:38 | friendly-scanner | VN | | 210.211.122.14 | | 207349 | 1611166586 | 75 | 2019-08-23 17:39:14 | Conaito | US | Buffalo | 96.8.115.122 | | 207758 | 2207850059 | 2 | 2019-08-23 07:04:37 | friendly-scanner | US | Atlanta | 131.153.30.75 | | 207804 | 3644668999 | 1 | 2019-08-22 11:35:07 | friendly-scanner | IT | Ponte San Pietro | 217.61.60.71 | | 208524 | 1066345266 | 1 | 2019-08-22 12:22:59 | friendly-scanner | US | Dallas | 63.143.35.50 | | 212570 | 1051564796 | 1 | 2019-08-22 16:38:40 | friendly-scanner | RU | Moscow | 62.173.154.252 | | 214517 | 1168277118 | 25 | 2019-08-23 06:00:47 | | US | Dallas | 69.162.126.126 | | 214658 | 3107280948 | 1 | 2019-08-22 18:58:40 | friendly-scanner | NL | | 185.53.88.52 | | 215069 | 2382630478 | 12911 | 2019-08-22 19:31:21 | Asterisk PBX | US | Provo | 142.4.14.78 | | 229501 | 3507576850 | 1 | 2019-08-22 22:57:06 | VnghmpQZ | US | | 209.17.96.18 | | 229709 | 1446618158 | 1 | 2019-08-22 23:53:04 | friendly-scanner | BY | Hrodna | 86.57.164.46 | | 229774 | 1051560609 | 1 | 2019-08-23 00:10:51 | friendly-scanner | RU | | 62.173.138.161 | | 229848 | 602425983 | 1 | 2019-08-23 01:44:50 | friendly-scanner | US | | 35.232.74.127 | | 229891 | 1051563810 | 1 | 2019-08-23 04:02:30 | friendly-scanner | RU | Moscow | 62.173.151.34 | | 229907 | 1551278113 | 1 | 2019-08-23 04:56:25 | vdjbTQHQ | LT | | 92.118.160.33 | | 230115 | 1168270182 | 1 | 2019-08-23 14:01:07 | friendly-scanner | US | Dallas | 69.162.99.102 | | 230116 | 87965622 | 31 | 2019-08-23 21:29:53 | pplsip | US | New York | 5.62.63.182 | | 230122 | 392083557 | 1 | 2019-08-23 14:31:38 | friendly-scanner | US | Buffalo | 23.94.184.101 | | 230125 | 1870059136 | 1 | 2019-08-23 15:01:48 | friendly-scanner | IN | | 111.118.214.128 | +--------+------------+-------+---------------------+----------------------------+---------+---------------------+-----------------------+ 172 rows in set (0.00 sec)